前言
上一篇文章,我们一起学习了 Shell
脚本中的 if
条件控制,以及多种条件判断,本篇文章我们一起来学习下剩余的流程控制语句。
流程控制语句
我们先来看下 Shell
中常见的流程控制:
- if…else:和所有语言的if…else…类型一样
- case…esac:与其他语言中的 switch … case 语句类似
- for:与其他语言中的 for 循环一样
- while:和其他语言的 while 循环一样
- until循环:与 while 的循环处理方式相反
- break:跳出循环
- continue:跳出本次循环
case…esac语句
和 if...fi
类似,Shell
中的分支选择以 case
开始,以 esac
结束,结束标志都为开始语句的单词的倒序输入。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
case $变量名 in
"值1")
程序1
;;
"值2")
程序2
;;
...
*)
都不成立,执行
;;
esac
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
[root@VM-0-5-centos ~]# vim case.sh
#!/bin/bash
read -t 30 -p "please input your age: " age
case $age in
18)
echo "18"
;;
20)
echo "20"
;;
*)
echo "others"
;;
esac
[root@VM-0-5-centos ~]# chmod 755 case.sh
[root@VM-0-5-centos ~]# ./case.sh
please input your age: 20
20
[root@VM-0-5-centos ~]# ./case.sh
please input your age: 15
others
[root@VM-0-5-centos ~]# ./case.sh
please input your age: 18
18
|
for循环
语法一
for
循环遍历整个列表进行循环,列表内可以是数字、字符串等。
1
2
3
4
|
for 变量 in 值1 值2 值3...
do
程序
done
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
# 累加示例
[root@VM-0-5-centos ~]# vim for_test.sh
#!/bin/bash
sum=0
for i in 1 2 3 4 5 6
do
sum=$(( $sum+$i ))
done
echo $sum
[root@VM-0-5-centos ~]# chmod 755 for_test.sh
[root@VM-0-5-centos ~]# ./for_test.sh
21
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
# 批量解压数据示例
[root@VM-0-5-centos ~]# vim example.sh
#!/bin/bash
ls *.tar.gz >> ls.log
for i in $(cat ls.log)
do
echo $i
tar -zxvf $i &>/dev/null
done
rm -rf ls.log
[root@VM-0-5-centos ~]# chmod 755 example.sh
[root@VM-0-5-centos ~]# ./example.sh
case.tar.gz
fot_test.tar.gz
[root@VM-0-5-centos ~]# ls
case.sh case.tar.gz example.sh for_test.sh fot_test.tar.gz
|
语法二
使用变量控制的方式,来进行 for
循环
1
2
3
4
|
for ((初始值;循环控制;变量变化))
do
程序
done
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
# 测试累加1到100
[root@VM-0-5-centos ~]# vim for_test_2.sh
#!/bin/bash
sum=0
for((i=0;i<=100;i++))
do
sum=$(($sum+i))
done
echo $sum
[root@VM-0-5-centos ~]# chmod 755 for_test_2.sh
[root@VM-0-5-centos ~]# ./for_test_2.sh
5050
|
while循环
while
循环,是在条件成立时进入循环
1
2
3
4
|
while [ 条件判断式 ]
do
程序
done
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
# 测试1加到100
[root@VM-0-5-centos ~]# vim while_test.sh
#!/bin/bash
sum=0
i=0
while [ $i -le 100 ]
do
sum=$(($sum+$i))
i=$(($i+1))
done
echo $sum
[root@VM-0-5-centos ~]# chmod 755 while_test.sh
[root@VM-0-5-centos ~]# ./while_test.sh
5050
|
until循环
until
在条件不成立时进入循环,条件成立则终止循环
1
2
3
4
|
until [ 条件判断式 ]
do
程序
done
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
# 测试1加到100
[root@VM-0-5-centos ~]# vim until_test.sh
#!/bin/bash
i=0
sum=0
until [ $i -gt 100 ]
do
sum=$(($sum+$i))
i=$(($i+1))
done
echo $sum
[root@VM-0-5-centos ~]# chmod 755 until_test.sh
[root@VM-0-5-centos ~]# ./until_test.sh
5050
|
break
和其他语言类似,break
用于跳出循环
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
# 当循环到10之后,跳出循环
[root@VM-0-5-centos ~]# vim break_test.sh
#!/bin/bash
for ((i=0;i<100;i++))
do
echo $i
if [ $i -eq 10 ]
then
break
fi
done
[root@VM-0-5-centos ~]# chmod 755 break_test.sh
[root@VM-0-5-centos ~]# ./break_test.sh
0
1
2
3
4
5
6
7
8
9
10
|
continue
和其他语言类似,continue
用于跳出当前循环
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
# 跳出循环,不打印3
[root@VM-0-5-centos ~]# vim continue_test.sh
#!/bin/bash
for ((i=0;i<5;i++))
do
if [ $i -eq 3 ]
then
continue
fi
echo $i
done
[root@VM-0-5-centos ~]# chmod 755 continue_test.sh
[root@VM-0-5-centos ~]# ./continue_test.sh
0
1
2
4
|
总结
本篇文章我们学习了 Shell
脚本中的剩余流程控制语句,包括以下几种:
-
if
-
case…esac
-
for
-
while
-
until
-
break
-
continue
学到这里,加上我们之前学习的 if 流程控制,条件判断、Bash中的变量类型、再加上更早之前的各种 Linux 命令,我们就可以编写简单的脚本去完成一些功能了。当然如果需要完成复杂功能的脚本,还需要我们去学习更多的 Linux 命令,在日积月累中完成蜕变。你必须非常努力,才能看起来毫不费力,一起 from zero to zero !!!
更多
微信公众号:CodePlayer
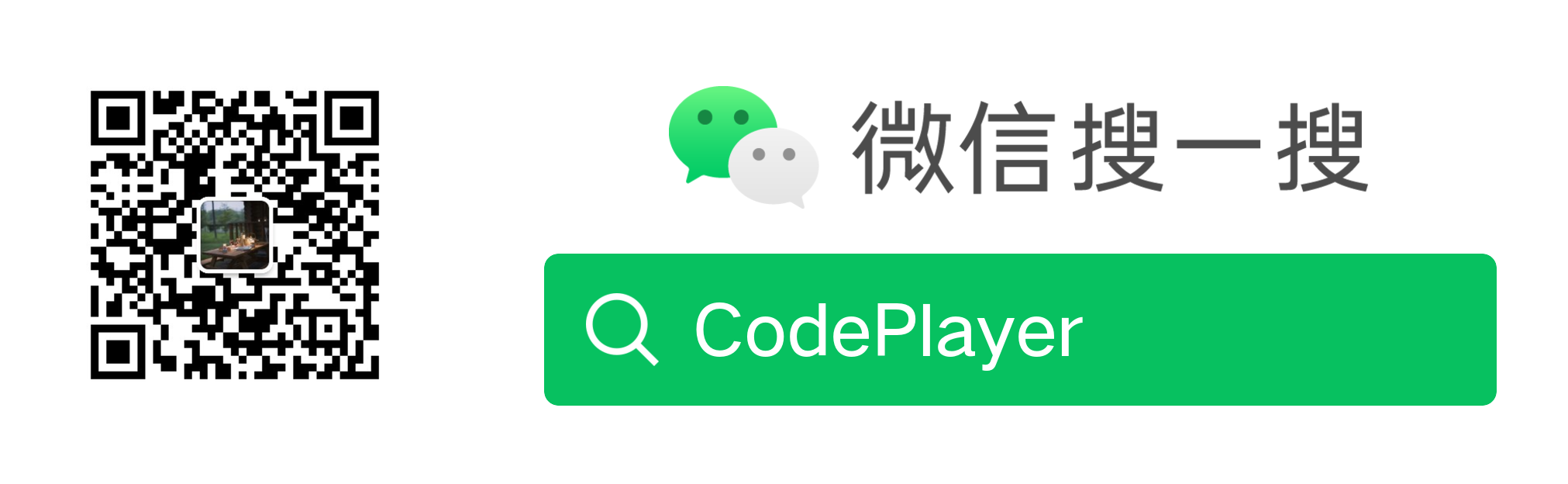